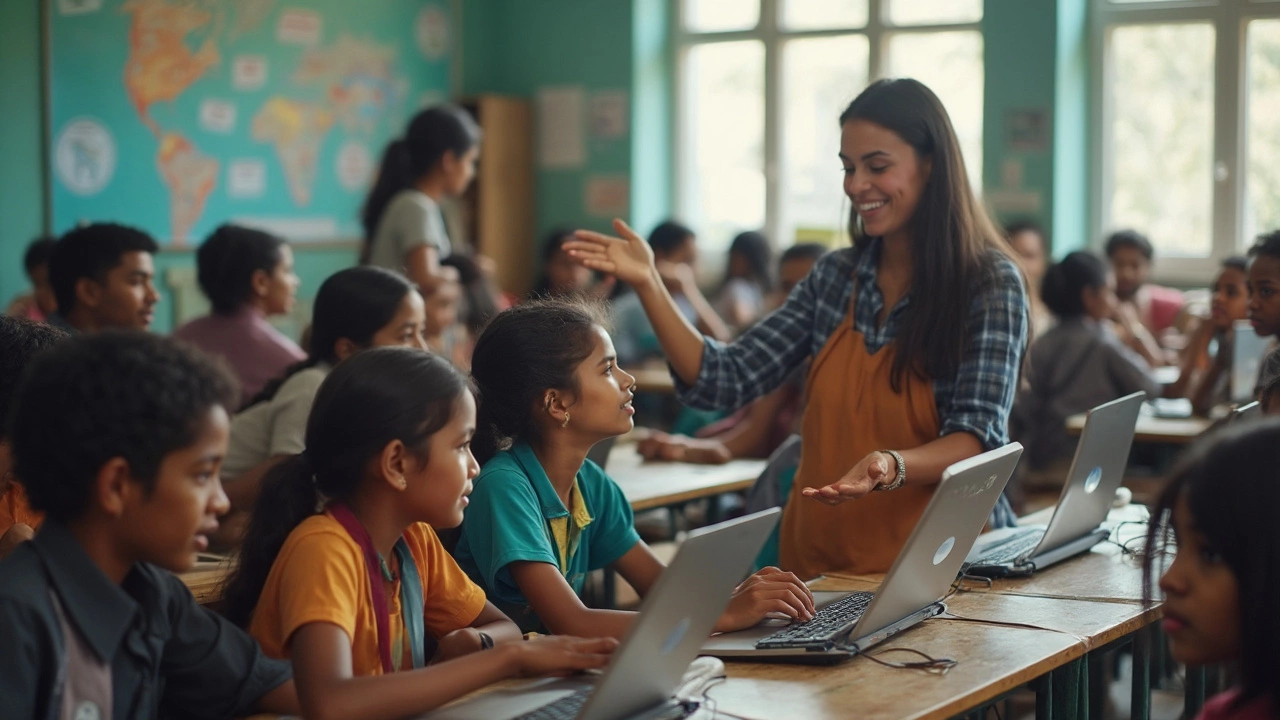
So, you're diving into the coding world, huh? It's like learning a new language, and like any language, it comes with its own set of rules and quirks. But don't worry—breaking it down into manageable steps can make the whole process way less scary. Let's start by understanding the problem. Before you type anything, get clear on what you're actually trying to solve. Think of it like planning a road trip. You wouldn't just hop in the car without knowing your destination, right?
Next up is planning your solution. This is all about figuring out the best way to get from point A to point B. Sketch out your plan, maybe draw a flowchart. The idea is to have a blueprint before you start building.
Once you've got a plan, it's time to start coding. This is where you're translating your solution into a language the computer can understand. It might feel slow at first, but hang in there. You're building something real!
- Understanding the Problem
- Planning the Solution
- Coding the Solution
- Testing the Code
- Debugging
- Refining and Enhancing
- Documentation
Understanding the Problem
Before you even start coding, it's crucial to wrap your head around the problem you're tackling. Imagine trying to solve a puzzle without knowing what picture you're creating—it's the same idea. Understanding the problem sets the foundation for everything else in the coding process.
So, how do you go about it? First, clearly define what needs solving. Is it a bug in an existing program? Or maybe you're creating an app from scratch? Whatever it is, spell it out. You can't hit a target if you don't know what you're aiming for.
Talk to the end-users if you can. What are their pain points? There's this great quote by Steve Jobs that rings true:
"You've got to start with the customer experience and work back toward the technology—not the other way around."Knowing what users need helps guide your solution.
Analyze the Requirements
Once you have the problem stated, dig into the details. Are there any constraints or requirements? Consider these things upfront to avoid headaches later. Jot down any assumptions or conditions that relate to your problem. It's like creating a map for your coding journey.
Additionally, breaking the problem into smaller parts can be a game-changer. Instead of one massive issue, you tackle a bunch of tiny challenges, making it all feel way more manageable.
Remember, this step is all about grasping what you're up against before jumping into the bright lights of coding. Take your time here, and your future self will thank you.
Planning the Solution
Alright, you've got a handle on the problem; now it's time to figure out the best way to solve it. Think of it like planning a big meal—you wouldn't start cooking without knowing what dishes you're making, right?
Planning the solution is all about laying down a roadmap. It's where you'll decide the approach and tools you'll use. Start by breaking the problem into smaller chunks. This makes it easier to tackle and helps you see the path more clearly.
Define Clear Steps
First things first, jot down the main tasks your code needs to accomplish. It helps set a direction and keeps you from veering off course. You can draft these in a step-by-step manner, like this:
- Identify inputs and outputs
- Outline the core functionality
- Determine necessary data structures
- Break large tasks into smaller, manageable ones
Choose Your Tools Wisely
Different problems require different tools. Need to handle data efficiently? Maybe a list or a dictionary will come in handy. Looking to design a user interface? Think about frameworks or libraries that fit the bill. Your toolbox is essential to coding, just like picking the right knife for chopping veggies.
Once your plan is sketched out, double-check it. Ask yourself, does it cover all the requirements? If something seems off, tweak it. It's better to mold the plan now before getting knee-deep in code.
Mock-Ups and Flowcharts
Visual aids can be a lifesaver. Crafting a flowchart helps in visualizing how data flows through the system. Even a rough mock-up of your UI can make things easier when you're deep in the coding trenches.
And remember, failing to plan is planning to fail. Set aside time to ensure your plan is solid. It sets the stage for the actual coding, and a strong plan often leads to strong code!
Coding the Solution
So, you've got your problem figured out and a plan drafted out. Awesome! Now, it's time to dive into coding. It's the moment where you transform those thoughts into lines of programming magic. Think of it as the building phase of constructing your blueprint into a real-world structure.
Setting Up Your Environment
First, make sure your coding environment is all set up. Install the necessary software and tools. If you're working in Python, make sure you've got an IDE, like PyCharm or VSCode. It's like picking the right set of tools for the job – a hammer won't do what a screwdriver can.
Writing the Code
Start typing! Follow the plan and don't rush. It’s crucial to keep your solution clean and understandable. Write functions for repetitive tasks. You might feel the itch to skip a bit, but sticking to your plan is key. Coding requires the right mix of precision and creativity.
Sticking with Best Practices
Keep your code tidy with good naming conventions and comments. This isn’t just for others – trust me, your future self will thank you when you revisit the code after a while. It's like leaving notes for why you took specific turns on your road trip.
Understanding Debugging Basics
You’re bound to hit a few snags along the way. Errors happen – it’s part of learning to code. Don’t freak out! Use debugging tools to find out what's going wrong and fix it. It's all part of developing your problem-solving skills.
Common Debugging Tools | Programming Language |
---|---|
Debugger | C++, Java |
Print Statements | Python, JavaScript |
Error Logs | PHP |
Remember, coding is as much about the journey as the destination. Take breaks, step back if needed, and remember why you started. You’re building something amazing one line at a time!
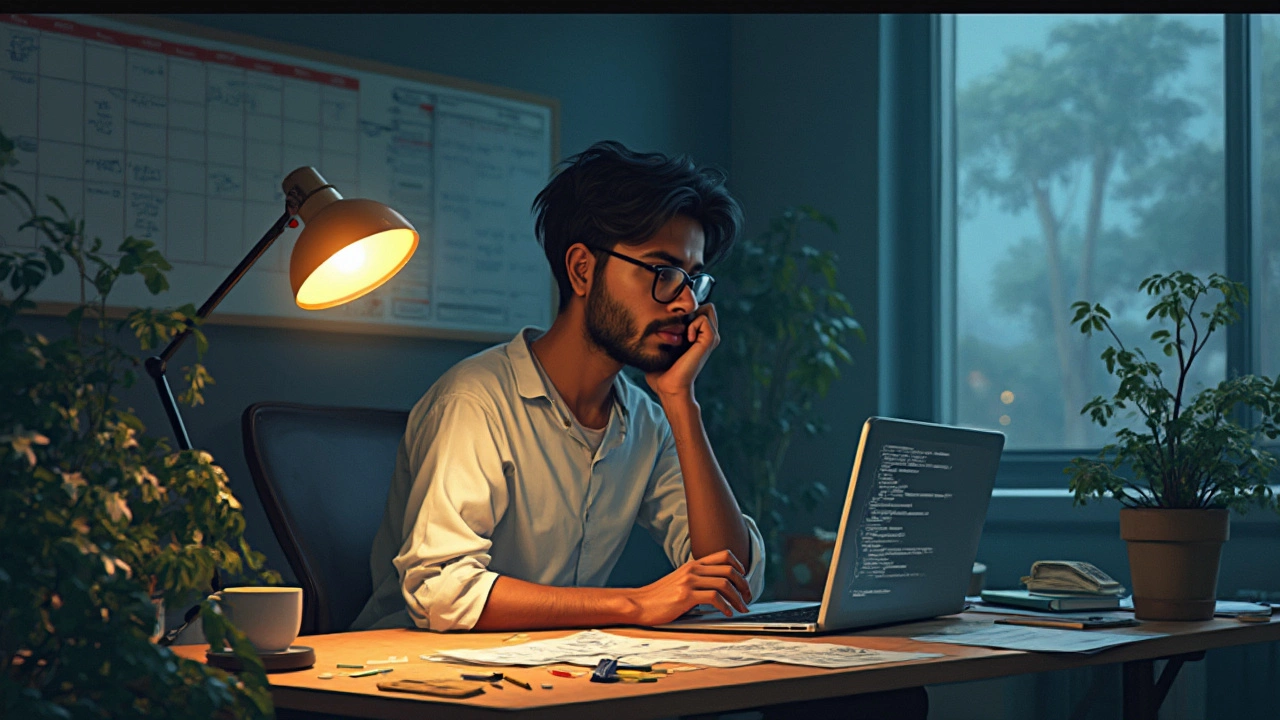
Testing the Code
Alright, so you've got your code written. But how do you know it actually works? That's where testing comes in. Think of it like baking a cake—you don't just throw it in the oven and hope for the best, right? You’ve gotta check if it rises, if it tastes good, and all that jazz. Similarly, testing the code is your way of checking if everything is working as expected.
Testing is all about validating your solution, making sure it does what it's supposed to do under different conditions. It's not just a one-time thing either. Testing is an ongoing process, happening throughout the coding process to catch bugs early before they morph into bigger problems.
Why Testing Matters
The importance of testing can't be stressed enough. We're talking about saving time and headaches down the road. Imagine finding out months later that there's a bug busting your software. It's way easier to fix stuff when it's fresh in your mind.
Also, testing helps you see where your code stands performance-wise. Is it running efficiently, or is it slow as molasses? Testing can give you these insights, helping you deliver a better product.
Types of Testing
- Unit Testing: This focuses on individual parts of your code, like functions or methods. It's like checking the ingredients of your cake separately before mixing.
- Integration Testing: Once you know the parts work, you need to check if they play nice together. Here, you're making sure your modules or services integrate without any hiccups.
- System Testing: Think of this as the final rehearsal. You test the entire coding solution as a whole to ensure everything works together perfectly.
- User Acceptance Testing (UAT): This is the user's turn to see if everything aligns with their needs and expectations.
Remember, no piece of software is perfect, and that's okay. The aim with testing is to reduce the number of bugs and make the code base as solid as possible. Got any cool testing tools you like? Share them with your coding buddies! Testing isn’t just a chore; it’s part of crafting something awesome.
Debugging
Alright, you've coded your heart out and hit run, but instead of that sweet end result, you're seeing unexpected outcomes or worst, an error. That's where debugging comes in. It's like detective work for your code, and it can be oddly satisfying once you get the hang of it.
Debugging is all about finding and fixing errors. Think of your code as a puzzle; sometimes pieces get misplaced. Your job is to figure out what's causing the problem and how to fix it without breaking everything else.
Why Debugging is Crucial
Even seasoned programmers spend a chunk of their time debugging. It's a crucial step because it ensures your code not only runs but runs well. Poor debugging can lead to software bugs that are not just annoying but potentially damaging for users.
Common Debugging Techniques
- Print Statements: Inserting print statements in your code is an old but reliable method to check the values of variables and the flow of your program.
- Debugging Tools: Use integrated development environments (IDEs) that come with built-in debugging tools. These let you step through your code line-by-line.
- Rubber Duck Debugging: Yes, talking to a rubber duck is an actual technique. By explaining your code out loud, you often find the error yourself.
- Unit Testing: Automated tests for individual parts of your code can catch bugs early. They might take time to write, but they save a lot of debugging time later on.
Statistics on Debugging
For those who enjoy numbers, a survey found that developers spend up to 50% of their coding time on debugging. That's like half your day! Knowing this might make it feel less frustrating when you're stuck, thinking of it as an integral part of the coding steps.
At the end of the day, debugging is your opportunity to learn more about how your code works (and sometimes doesn't work). Embrace it. You'll become a better coder with every bug you squash!
Refining and Enhancing
Okay, so you've got your code working. That's awesome! But the journey doesn't end with code that just works. This is where refining and enhancing come into play. Think of it like revising a draft. Now's your chance to make those tweaks that turn good code into great code.
First off, let's talk about optimization. You're looking to make your code run faster, use less memory, and just be more efficient overall. It involves finding any repetitive tasks and seeing how you can simplify them. Often, you'll find that using built-in functions or methods can streamline processes significantly.
Cleanup Time
Next, you'll want to clean up your code. This might seem tedious, but it's super important. Remove any commented-out code that's no longer needed, and make sure everything is well-indented and easy to read. Other developers (or future you) will thank you for this.
Refinement also means making the user experience better. Maybe you've found a more intuitive way to navigate through a task, or you've thought of an additional feature that users will love. This is the time to add that extra layer of polish.
Seize The Enhancement Opportunities
Here's another neat layer: input from users and stakeholders. Sometimes, what looks perfect to us might not fully align with what users need. Getting feedback helps you understand what changes or enhancements are necessary.
And hey, you might want to check out some stats if advancements really excite you. Did you know that enhanced code can often cut runtime by 30% depending on the complexity of tasks and project requirements? Keeping efficiency in mind, try incorporating methods or libraries known for their performance improvements.
Making all these improvements involves experimenting and maybe breaking things a little as you go, but it's all part of the process. Refining and enhancing make sure your coding not only solves the problem but does so in the best way possible.
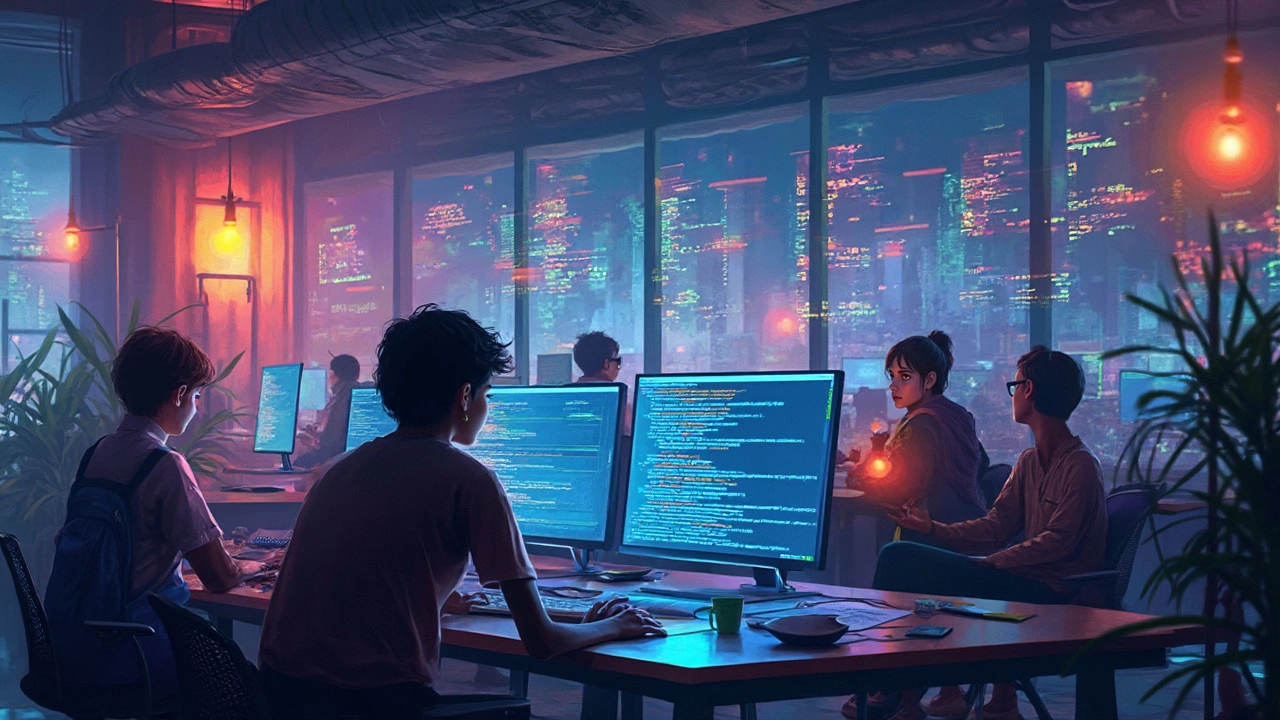
Documentation
Alright, you've got your code running smoothly—woohoo! But hold on, you're not quite done yet. Enter the world of documentation. Think of it as writing a diary entry but for your code, so you or someone else will get what you've done weeks, months, or years down the line. It's about leaving a trail so no one's left scratching their head trying to figure out a complex codebase.
So, what does good documentation consist of? Let's break it down. First, make sure you comment directly in your code. Comments are like your code's footnotes, explaining the why behind your logical leaps.
Code Comments
- Explain complex logic with simple language.
- Make them concise but clear. No need for essays.
- Avoid stating the obvious. Comment the why, not the what.
User Documentation
This is crucial if your code is going to be used by someone other than yourself—which, hint, it usually is. This might be a README file in your project's root folder, outlining what's what and how to get started.
- Start by explaining the project’s purpose and its key features.
- Include installation steps. Make it a breeze to get going.
- Add examples on how to run or use the code.
Good documentation separates pro coders from the rest. Plus, it can save hours of work down the road. You wouldn't leave a puzzle without clues for someone else to solve, right?